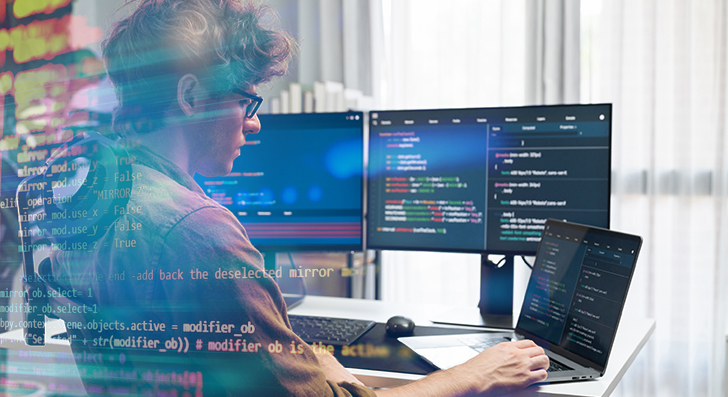
Scalability indicates your software can deal with growth—extra end users, a lot more data, and more targeted visitors—with no breaking. Like a developer, creating with scalability in your mind will save time and stress afterwards. Right here’s a transparent and functional guide to assist you to get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on later on—it should be portion of your system from the beginning. Lots of programs are unsuccessful after they mature quickly for the reason that the initial structure can’t manage the extra load. Like a developer, you might want to Feel early regarding how your system will behave under pressure.
Get started by creating your architecture being flexible. Prevent monolithic codebases where almost everything is tightly related. Rather, use modular layout or microservices. These styles break your app into more compact, independent elements. Just about every module or service can scale on its own without having influencing The complete system.
Also, take into consideration your databases from working day 1. Will it need to have to manage one million users or simply a hundred? Select the appropriate form—relational or NoSQL—depending on how your knowledge will improve. Approach for sharding, indexing, and backups early, even if you don’t need them however.
Yet another critical place is to stop hardcoding assumptions. Don’t create code that only operates beneath recent problems. Contemplate what would transpire If the person foundation doubled tomorrow. Would your app crash? Would the databases slow down?
Use style and design designs that assist scaling, like information queues or celebration-driven techniques. These aid your app cope with additional requests devoid of finding overloaded.
If you Create with scalability in mind, you're not just preparing for fulfillment—you might be reducing future problems. A very well-prepared program is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the proper Database
Deciding on the appropriate database is a critical Section of developing scalable applications. Not all databases are designed the exact same, and using the Incorrect you can sluggish you down or even bring about failures as your app grows.
Get started by knowledge your info. Is it extremely structured, like rows inside of a table? If Certainly, a relational database like PostgreSQL or MySQL is a good in good shape. These are generally powerful with interactions, transactions, and consistency. Additionally they assist scaling methods like examine replicas, indexing, and partitioning to deal with extra site visitors and details.
Should your data is much more adaptable—like user exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured info and will scale horizontally much more quickly.
Also, contemplate your examine and create designs. Are you presently performing a great deal of reads with much less writes? Use caching and read replicas. Have you been managing a heavy publish load? Take a look at databases that may take care of superior write throughput, and even celebration-centered data storage methods like Apache Kafka (for short term facts streams).
It’s also intelligent to Feel forward. You might not will need Highly developed scaling features now, but choosing a database that supports them implies you gained’t need to have to change afterwards.
Use indexing to hurry up queries. Avoid unnecessary joins. Normalize or denormalize your information according to your accessibility designs. And often keep an eye on database functionality while you improve.
To put it briefly, the ideal databases will depend on your application’s framework, pace wants, And the way you count on it to expand. Consider time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, every small hold off provides up. Inadequately prepared code or unoptimized queries can slow down performance and overload your system. That’s why it’s imperative that you Make productive logic from the start.
Start by producing clear, straightforward code. Keep away from repeating logic and remove just about anything unwanted. Don’t select the most complex Alternative if an easy 1 is effective. Maintain your functions small, targeted, and simple to check. Use profiling equipment to locate bottlenecks—sites the place your code requires far too extended to operate or employs too much memory.
Upcoming, examine your database queries. These generally slow points down greater than the code alone. Make certain Just about every query only asks for the information you actually need to have. Avoid Decide on *, which fetches every little thing, and in its place pick unique fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across huge tables.
For those who discover the exact same info staying asked for repeatedly, use caching. Keep the effects temporarily employing instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application additional economical.
Remember to test with huge datasets. Code and queries that operate high-quality with a hundred documents might crash if they have to take care of one million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when needed. These actions aid your application remain easy and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage additional people plus much more targeted visitors. If all the things goes as a result of a person server, it will eventually quickly turn into a bottleneck. That’s wherever load balancing and caching come in. Both of these applications enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server carrying out each of the function, the load balancer routes consumers to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to set up.
Caching is about storing details quickly so it may be reused rapidly. When buyers request exactly the same information and facts once again—like a product site or even a profile—you don’t have to fetch it within the database every time. It is possible to serve it from the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for quickly obtain.
2. Shopper-side caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lowers database load, increases speed, and can make your app far more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does adjust.
To put it briefly, load balancing and caching are straightforward but potent instruments. Together, they help your application tackle much more end users, continue to be quick, and Get well from complications. If you plan to expand, you require both.
Use Cloud and Container Equipment
To develop scalable programs, you may need applications that permit your app expand simply. That’s where by cloud platforms and containers come in. They give you versatility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and expert services as you would like them. You don’t have to purchase hardware or guess long term capability. When site visitors will increase, it is possible to insert additional methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also offer services like managed databases, storage, load balancing, and stability applications. You could deal with setting up your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one device. This causes it to be straightforward to move your application amongst environments, out of your notebook to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single element of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale components independently, which happens to be great for general performance and dependability.
In short, employing cloud and container tools suggests you are able to scale speedy, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow with no restrictions, commence applying these resources early. They help save time, reduce chance, and assist you remain centered on setting up, not fixing.
Keep an eye on Every thing
In case you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, place troubles early, and make improved decisions as your app grows. It’s a essential Component of setting up scalable methods.
Commence by monitoring primary metrics like CPU use, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this knowledge.
Don’t just keep an eye on your website servers—watch your application much too. Regulate how much time it takes for users to load pages, how often errors occur, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes previously mentioned a limit or perhaps a service goes down, you should get notified immediately. This helps you take care of challenges rapid, typically ahead of consumers even discover.
Checking is likewise valuable once you make adjustments. In case you deploy a fresh function and find out a spike in problems or slowdowns, you'll be able to roll it back right before it brings about actual damage.
As your application grows, site visitors and information maximize. With no monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it works properly, even stressed.
Ultimate Views
Scalability isn’t just for significant organizations. Even compact apps will need a strong Basis. By designing meticulously, optimizing sensibly, and using the suitable tools, you may build apps that improve easily without the need of breaking under pressure. Start off small, Feel major, and Develop sensible.