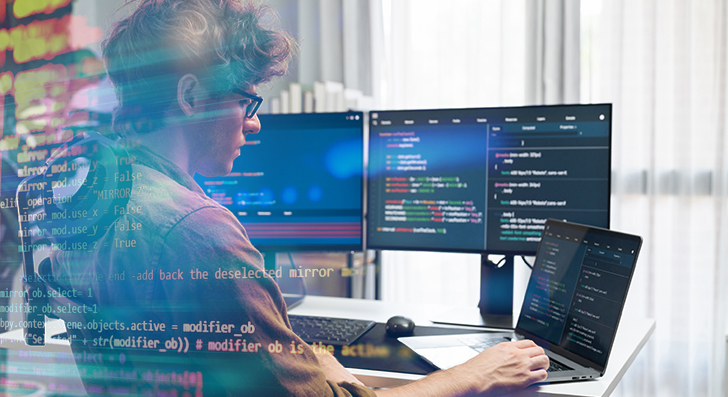
Scalability implies your software can take care of progress—much more buyers, additional knowledge, and more targeted visitors—devoid of breaking. Being a developer, developing with scalability in your mind saves time and worry later on. Here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be part of your respective strategy from the start. Numerous apps fail if they develop rapid due to the fact the original layout can’t handle the extra load. For a developer, you have to Assume early about how your technique will behave stressed.
Start off by designing your architecture for being adaptable. Avoid monolithic codebases the place all the things is tightly connected. Alternatively, use modular design or microservices. These designs crack your app into smaller sized, impartial pieces. Every module or provider can scale By itself without the need of affecting The full procedure.
Also, consider your database from day just one. Will it have to have to handle a million consumers or merely a hundred? Choose the suitable kind—relational or NoSQL—based upon how your details will develop. Program for sharding, indexing, and backups early, Even though you don’t have to have them however.
A further crucial position is to stop hardcoding assumptions. Don’t produce code that only is effective under present situations. Think of what would transpire Should your consumer foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style patterns that help scaling, like information queues or function-pushed units. These assistance your application cope with extra requests without obtaining overloaded.
Whenever you Develop with scalability in mind, you're not just making ready for fulfillment—you happen to be minimizing potential headaches. A effectively-planned method is easier to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Databases
Picking out the appropriate database is a critical Element of constructing scalable programs. Not all databases are built a similar, and utilizing the Incorrect you can sluggish you down or even bring about failures as your application grows.
Commence by understanding your facts. Could it be highly structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb suit. These are typically powerful with interactions, transactions, and consistency. In addition they assistance scaling procedures like go through replicas, indexing, and partitioning to take care of far more traffic and facts.
When your data is much more adaptable—like user action logs, product catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with large volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more quickly.
Also, think about your read through and generate patterns. Will you be doing a lot of reads with much less writes? Use caching and browse replicas. Will you be managing a hefty publish load? Take a look at databases that can manage significant generate throughput, or perhaps function-based information storage techniques like Apache Kafka (for momentary details streams).
It’s also smart to Believe forward. You may not need to have State-of-the-art scaling features now, but choosing a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your data based on your accessibility patterns. And constantly watch databases effectiveness when you improve.
Briefly, the appropriate databases will depend on your application’s framework, pace desires, And just how you assume it to increase. Just take time to choose properly—it’ll save a lot of trouble afterwards.
Enhance Code and Queries
Rapidly code is essential to scalability. As your application grows, each and every tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Make productive logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most sophisticated solution if a straightforward a person performs. Keep your capabilities quick, focused, and straightforward to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes as well lengthy to operate or makes use of too much memory.
Following, take a look at your databases queries. These frequently gradual items down much more than the code by itself. Be certain Every single query only asks for the info you actually need to have. Steer clear of Pick out *, which fetches every thing, and as a substitute choose precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, especially across substantial tables.
In the event you detect the same knowledge remaining requested over and over, use caching. Retail store the results briefly working with tools like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your app a lot more productive.
Make sure to test with huge datasets. Code and queries that operate great with a hundred records may crash after they have to manage one million.
Briefly, scalable applications are speedy applications. Keep the code tight, your queries lean, and use caching when necessary. These methods support your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage additional people plus more targeted visitors. If every little thing goes by means of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors throughout several servers. As opposed to a single server performing all of the work, the load balancer routes buyers to unique servers based upon availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details briefly so it may be reused immediately. When people request the same facts once again—like an item site or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) shops details in memory for rapidly obtain.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app far more efficient.
Use caching for things which don’t improve usually. And usually ensure that your cache is updated when knowledge does improve.
In brief, load balancing and caching are very simple but potent instruments. Together, they help your application tackle much more people, continue to be quick, and Get better from issues. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need applications that let your app expand simply. That’s where by cloud platforms and containers come in. They give you versatility, minimize set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess foreseeable future ability. When targeted traffic boosts, you may increase much more sources with only a few clicks or instantly making use of automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You'll be able to concentrate on developing your app in lieu of running infrastructure.
Containers are A different key Software. A container offers your app and every thing it must operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from the laptop computer towards the cloud, without the need of surprises. Docker is the preferred Software for this.
Whenever your app takes advantage of a number of containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and recovery. If 1 section of the app crashes, it restarts it quickly.
Containers also ensure it is easy to individual elements of your application into companies. You are able to update or scale sections independently, and that is great for general performance and dependability.
To put it briefly, employing cloud and container tools signifies you are able to scale rapid, deploy effortlessly, and Get well rapidly when challenges occur. If you prefer your app to increase without boundaries, start making use of these tools early. They preserve time, cut down threat, and assist you stay focused on making, not fixing.
Check Anything
In the event you don’t keep an eye on your software, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location issues early, and make superior conclusions as your app grows. It’s a crucial Section of making scalable systems.
Begin by tracking standard metrics like CPU use, memory, disk House, and reaction time. These show you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep watch over how much time it will require for buyers to load internet pages, how frequently faults materialize, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a support goes down, you ought to get notified right away. This assists you correct troubles quickly, frequently prior to users even see.
Checking can be beneficial whenever you make changes. For those who deploy a different characteristic and see a spike in faults or slowdowns, you may roll it back again before it results in true injury.
As your application grows, website traffic and info increase. Devoid of checking, you’ll skip indications of difficulties till it’s much too late. But with the best tools set here up, you remain on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it really works properly, even stressed.
Ultimate Views
Scalability isn’t just for major businesses. Even smaller apps need a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you may Develop applications that mature easily devoid of breaking stressed. Commence smaller, think massive, and Establish intelligent.